EC2 is one of the most popular web services provided by AWS that provides resizable compute capacity in the cloud. EC2 instances can be started and stopped on demand, which allows you to pay only for the resources you need.
In this blog post, we will discuss how to write a simple script to stop your EC2 instances at night when your workload is minimal to save costs.
When you launch an EC2 instance, you are charged for the time the instance is running. If you have instances that are not in use, stopping them will help reduce your costs.
Additionally, stopping your instances at night when your workload is minimal will help you save money on your AWS bill. EC2 instances can be stopped and started again at any time, so you can use this feature to save your AWS cost.
To set up EC2 Auto start and stop scripts with Lambda, you’ll need to follow a few key steps:
Step 1: Create IAM Role for Lambda functions
Log into the AWS Account >> IAM >> Roles >> Create role >> choose lambda in use case >> Assign AmazonEC2FullAccess, CloudWatchLogsFullAccess policies >> assign role name >> create role
Step 2: Create Lambda Function for Auto Start
- Log in to AWS Console with an IAM user.
- Navigate to Lambda Service and select it.
- Click on create function.
- Enter function name – EC2AutoStart
- Select runtime environment – Python3.9
- Select Architecture *86_64
- Select Use an existing role.
- In the existing role select the IAM role we have created earlier in step 1
- Click on Create function
- Copy and paste the code
- Do necessary changes like instance id, region etc.
import boto3
region = 'ap-south-1'
Instances = ['#your_instance_id','#your_instance_id']
ec2 = boto3.client('ec2', region_name=region)
def lambda_handler(event, context):
ec2.start_instances(InstanceIds=Instances)
print('started instances: ' + str(Instances))
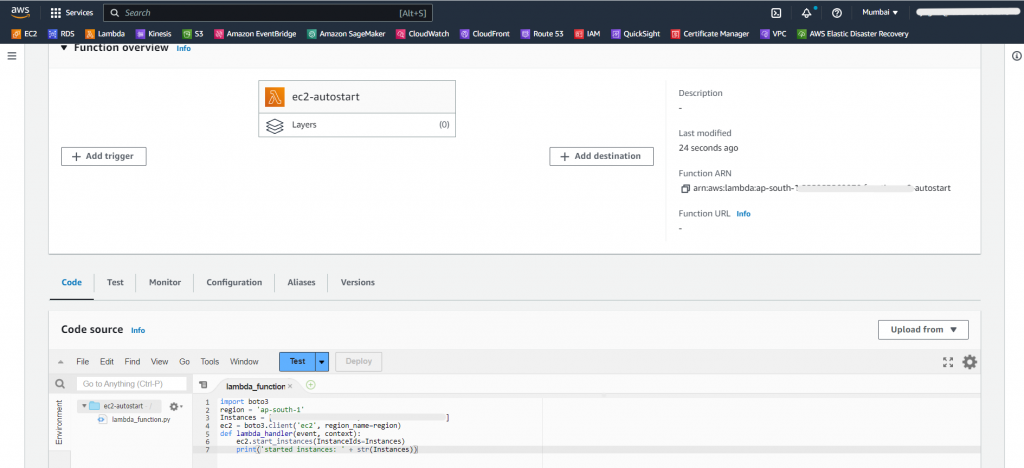
- Click on Deploy
- Click on configuration and select general configuration
- Click on edit
- Set time out from 3 sec to 3 min.
- Click on Save.
Step 3: Create Lambda Function for Auto Stop
- Log in to AWS Console with an IAM user.
- Navigate to Lambda Service.
- Click on create function.
- Enter function name – [EC2AutoStop]
- Select runtime environment – Python3.9.
- Select Architecture *86_64
- Select Use an existing role.
- In existing role select the IAM role we have created earlier in step1
- Click on Create function
- Copy and paste the code
- Do necessary changes like instance id, region etc.
import boto3
region = 'ap-south-1'
Instances = ['#your_instance_id','#your_instance_id']
ec2 = boto3.client('ec2', region_name=region)
def lambda_handler(event, context):
ec2.stop_instances(InstanceIds=Instances)
print('stopped instances: ' + str(Instances))
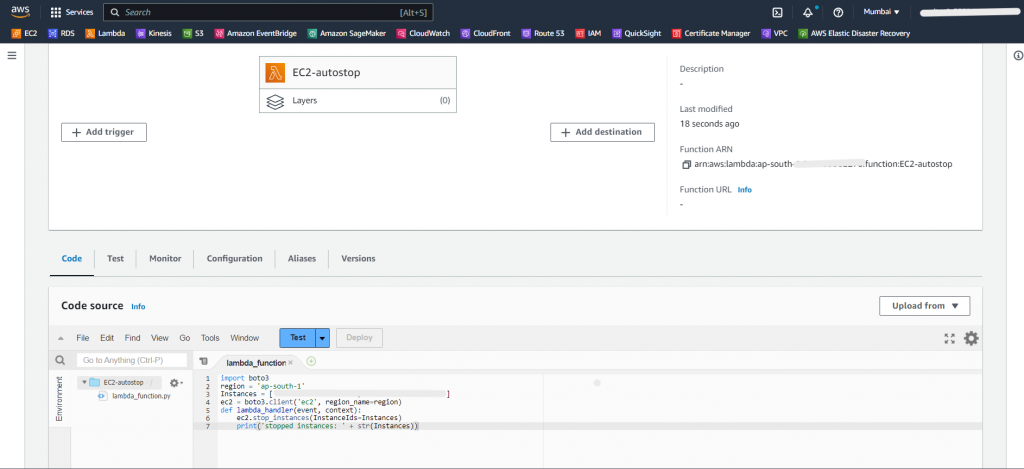
- Click on Deploy.
- Click on configuration select general configuration
- Click on edit
- Set time out from 3 sec to 3 min.
- Click on Save
Step 4: Create an Event Bridge Rule to start trigger lambda
- Log in to AWS Console with an IAM user.
- Navigate to EventBridge Service >> rules.
- Click on create the rule
- Enter rule name – [EC2AutoStart]
- Enter a description for rule
- Select Rule type – schedule >>continue to create rule
- Enter cron for when will it trigger lambda function for EC2 Auto start
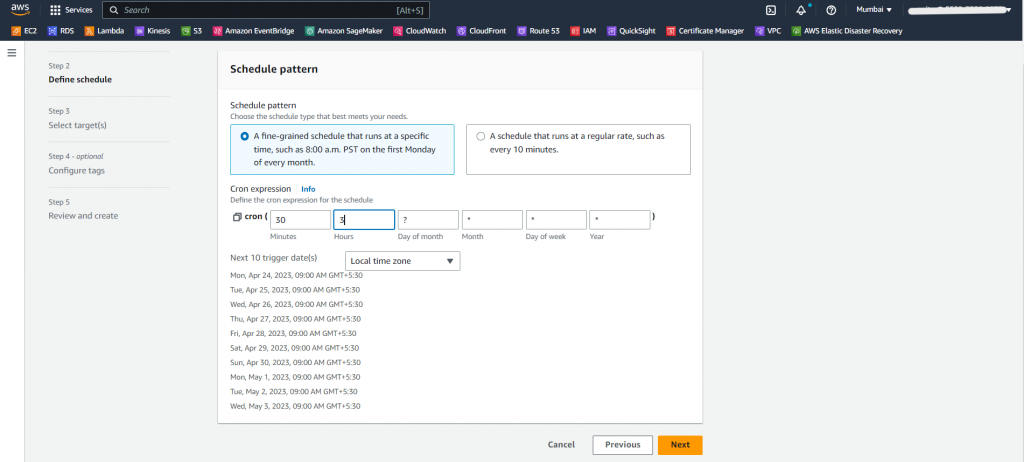
- Click on Next.
- Select a target Lambda function
- Select function – [EC2AutoStart]
- Click on next, again click on next
- Review and click on create rule
Step 5: Create an Event Bridge Rule to trigger lambda
- Log in to AWS Console with an IAM user.
- Navigate to EventBridge Service.
- Click on create the rule
- Enter rule name – [EC2AutoStop].
- Enter a description for tule
- Select Rule type – schedule >>continue to create rule
- Enter cron for when will it trigger lambda function for EC2 Auto stop
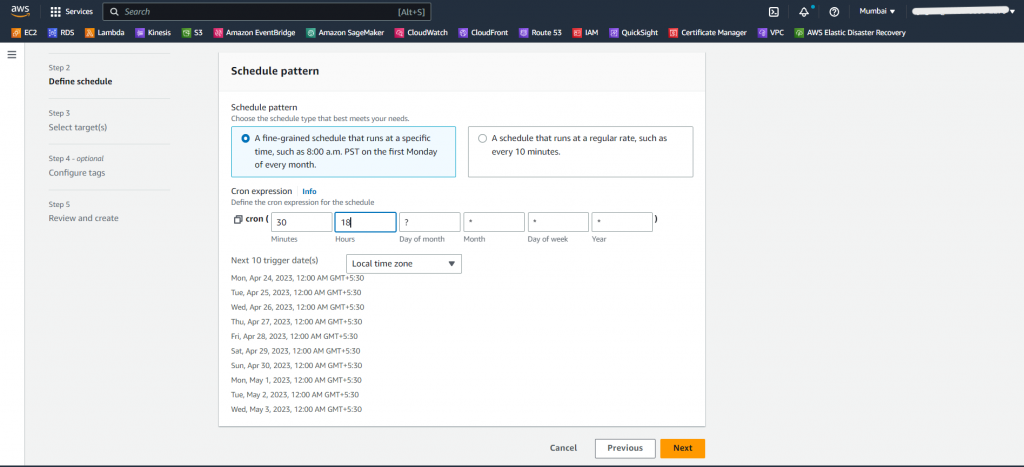
- Click on Next.
- Select a target Lambda function
- Select function – [EC2AutoStop]
- Click on next, again click on next
- Review and click on create rule
Conclusion
Stopping your EC2 instances at night when your workload is minimal is a simple way to save money on your AWS bill. By writing a simple script, you can automate the process of stopping your instances, making it easier to manage your infrastructure. With this script in place, you can optimize your AWS.